Implement a program in Matlab for LU decomposition with pivoting
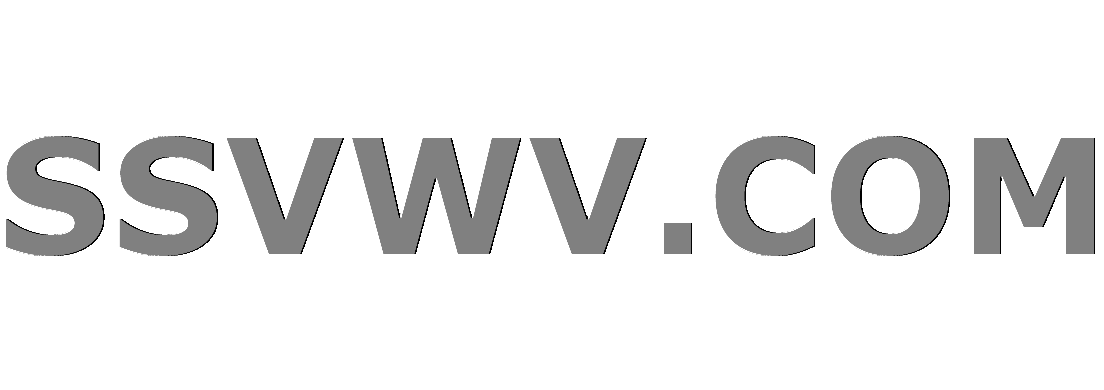
Multi tool use
$begingroup$
I need to write a program to solve matrix equations Ax=b where A is an nxn matrix, and b is a vector with n entries using LU decomposition. Unfortunately I'm not allowed to use any prewritten codes in Matlab. I am having problems with the first part of my code where i decompose the matrix in to an upper and lower matrix.
I have written the following code, and cannot work out why it is giving me all zeros on the diagonal in my lower matrix. Any improvements would be greatly appreciated.
function[L R]=LR2(A)
%Decomposition of Matrix AA: A = L R
z=size(A,1);
L=zeros(z,z);
R=zeros(z,z);
for i=1:z
% Finding L
for k=1:i-1
L(i,k)=A(i,k);
for j=1:k-1
L(i,k)= L(i,k)-L(i,j)*R(j,k);
end
L(i,k) = L(i,k)/R(k,k);
end
% Finding R
for k=i:z
R(i,k) = A(i,k);
for j=1:i-1
R(i,k)= R(i,k)-L(i,j)*R(j,k);
end
end
end
R
L
end
I know that i could simply assign all diagonal components of L to be 1, but would like to understand what the problem is with my program!
I am also wondering how to change this program to include pivoting. I understand I need to say that if a diagonal element is equal to zero something needs to be changed. How would I go about this?
Thanks in advance!
numerical-methods computer-science math-software matlab
$endgroup$
add a comment |
$begingroup$
I need to write a program to solve matrix equations Ax=b where A is an nxn matrix, and b is a vector with n entries using LU decomposition. Unfortunately I'm not allowed to use any prewritten codes in Matlab. I am having problems with the first part of my code where i decompose the matrix in to an upper and lower matrix.
I have written the following code, and cannot work out why it is giving me all zeros on the diagonal in my lower matrix. Any improvements would be greatly appreciated.
function[L R]=LR2(A)
%Decomposition of Matrix AA: A = L R
z=size(A,1);
L=zeros(z,z);
R=zeros(z,z);
for i=1:z
% Finding L
for k=1:i-1
L(i,k)=A(i,k);
for j=1:k-1
L(i,k)= L(i,k)-L(i,j)*R(j,k);
end
L(i,k) = L(i,k)/R(k,k);
end
% Finding R
for k=i:z
R(i,k) = A(i,k);
for j=1:i-1
R(i,k)= R(i,k)-L(i,j)*R(j,k);
end
end
end
R
L
end
I know that i could simply assign all diagonal components of L to be 1, but would like to understand what the problem is with my program!
I am also wondering how to change this program to include pivoting. I understand I need to say that if a diagonal element is equal to zero something needs to be changed. How would I go about this?
Thanks in advance!
numerical-methods computer-science math-software matlab
$endgroup$
2
$begingroup$
You have assigned $L$ to be a zero matrix and the for loop for $i^{th}$ row of $L$ runs from the first column to the $(i-1)^{th}$ column. The entry $L(i,i)$ is still left unchanged. So the diagonal entries still remain zero.
$endgroup$
– user17762
Jan 8 '11 at 22:29
$begingroup$
@Sivaram so would it be "cheating" to simply assign all of my L(i,i)s to be 1? Thanks alot :)
$endgroup$
– Lucy Marshall
Jan 8 '11 at 22:32
1
$begingroup$
No it wouldn't. The best way is to decompose $L$ and $U$ directly in $A$. Since you know there will be ones on the diagonal, for huge matrix dimensions saving the previous known zeros and ones is a waste of memory space.
$endgroup$
– TheWaveLad
Jun 2 '14 at 20:18
add a comment |
$begingroup$
I need to write a program to solve matrix equations Ax=b where A is an nxn matrix, and b is a vector with n entries using LU decomposition. Unfortunately I'm not allowed to use any prewritten codes in Matlab. I am having problems with the first part of my code where i decompose the matrix in to an upper and lower matrix.
I have written the following code, and cannot work out why it is giving me all zeros on the diagonal in my lower matrix. Any improvements would be greatly appreciated.
function[L R]=LR2(A)
%Decomposition of Matrix AA: A = L R
z=size(A,1);
L=zeros(z,z);
R=zeros(z,z);
for i=1:z
% Finding L
for k=1:i-1
L(i,k)=A(i,k);
for j=1:k-1
L(i,k)= L(i,k)-L(i,j)*R(j,k);
end
L(i,k) = L(i,k)/R(k,k);
end
% Finding R
for k=i:z
R(i,k) = A(i,k);
for j=1:i-1
R(i,k)= R(i,k)-L(i,j)*R(j,k);
end
end
end
R
L
end
I know that i could simply assign all diagonal components of L to be 1, but would like to understand what the problem is with my program!
I am also wondering how to change this program to include pivoting. I understand I need to say that if a diagonal element is equal to zero something needs to be changed. How would I go about this?
Thanks in advance!
numerical-methods computer-science math-software matlab
$endgroup$
I need to write a program to solve matrix equations Ax=b where A is an nxn matrix, and b is a vector with n entries using LU decomposition. Unfortunately I'm not allowed to use any prewritten codes in Matlab. I am having problems with the first part of my code where i decompose the matrix in to an upper and lower matrix.
I have written the following code, and cannot work out why it is giving me all zeros on the diagonal in my lower matrix. Any improvements would be greatly appreciated.
function[L R]=LR2(A)
%Decomposition of Matrix AA: A = L R
z=size(A,1);
L=zeros(z,z);
R=zeros(z,z);
for i=1:z
% Finding L
for k=1:i-1
L(i,k)=A(i,k);
for j=1:k-1
L(i,k)= L(i,k)-L(i,j)*R(j,k);
end
L(i,k) = L(i,k)/R(k,k);
end
% Finding R
for k=i:z
R(i,k) = A(i,k);
for j=1:i-1
R(i,k)= R(i,k)-L(i,j)*R(j,k);
end
end
end
R
L
end
I know that i could simply assign all diagonal components of L to be 1, but would like to understand what the problem is with my program!
I am also wondering how to change this program to include pivoting. I understand I need to say that if a diagonal element is equal to zero something needs to be changed. How would I go about this?
Thanks in advance!
numerical-methods computer-science math-software matlab
numerical-methods computer-science math-software matlab
edited Jan 9 '11 at 0:52
Aryabhata
69.9k6156246
69.9k6156246
asked Jan 8 '11 at 22:23
Lucy MarshallLucy Marshall
1471212
1471212
2
$begingroup$
You have assigned $L$ to be a zero matrix and the for loop for $i^{th}$ row of $L$ runs from the first column to the $(i-1)^{th}$ column. The entry $L(i,i)$ is still left unchanged. So the diagonal entries still remain zero.
$endgroup$
– user17762
Jan 8 '11 at 22:29
$begingroup$
@Sivaram so would it be "cheating" to simply assign all of my L(i,i)s to be 1? Thanks alot :)
$endgroup$
– Lucy Marshall
Jan 8 '11 at 22:32
1
$begingroup$
No it wouldn't. The best way is to decompose $L$ and $U$ directly in $A$. Since you know there will be ones on the diagonal, for huge matrix dimensions saving the previous known zeros and ones is a waste of memory space.
$endgroup$
– TheWaveLad
Jun 2 '14 at 20:18
add a comment |
2
$begingroup$
You have assigned $L$ to be a zero matrix and the for loop for $i^{th}$ row of $L$ runs from the first column to the $(i-1)^{th}$ column. The entry $L(i,i)$ is still left unchanged. So the diagonal entries still remain zero.
$endgroup$
– user17762
Jan 8 '11 at 22:29
$begingroup$
@Sivaram so would it be "cheating" to simply assign all of my L(i,i)s to be 1? Thanks alot :)
$endgroup$
– Lucy Marshall
Jan 8 '11 at 22:32
1
$begingroup$
No it wouldn't. The best way is to decompose $L$ and $U$ directly in $A$. Since you know there will be ones on the diagonal, for huge matrix dimensions saving the previous known zeros and ones is a waste of memory space.
$endgroup$
– TheWaveLad
Jun 2 '14 at 20:18
2
2
$begingroup$
You have assigned $L$ to be a zero matrix and the for loop for $i^{th}$ row of $L$ runs from the first column to the $(i-1)^{th}$ column. The entry $L(i,i)$ is still left unchanged. So the diagonal entries still remain zero.
$endgroup$
– user17762
Jan 8 '11 at 22:29
$begingroup$
You have assigned $L$ to be a zero matrix and the for loop for $i^{th}$ row of $L$ runs from the first column to the $(i-1)^{th}$ column. The entry $L(i,i)$ is still left unchanged. So the diagonal entries still remain zero.
$endgroup$
– user17762
Jan 8 '11 at 22:29
$begingroup$
@Sivaram so would it be "cheating" to simply assign all of my L(i,i)s to be 1? Thanks alot :)
$endgroup$
– Lucy Marshall
Jan 8 '11 at 22:32
$begingroup$
@Sivaram so would it be "cheating" to simply assign all of my L(i,i)s to be 1? Thanks alot :)
$endgroup$
– Lucy Marshall
Jan 8 '11 at 22:32
1
1
$begingroup$
No it wouldn't. The best way is to decompose $L$ and $U$ directly in $A$. Since you know there will be ones on the diagonal, for huge matrix dimensions saving the previous known zeros and ones is a waste of memory space.
$endgroup$
– TheWaveLad
Jun 2 '14 at 20:18
$begingroup$
No it wouldn't. The best way is to decompose $L$ and $U$ directly in $A$. Since you know there will be ones on the diagonal, for huge matrix dimensions saving the previous known zeros and ones is a waste of memory space.
$endgroup$
– TheWaveLad
Jun 2 '14 at 20:18
add a comment |
3 Answers
3
active
oldest
votes
$begingroup$
For backward and forward elimination I used
% Now use a vector y to solve 'Ly=b'
for j=1:z
for k=1:j-1
b(j)=b(j)-L(j,k)*b(k);
end;
b(j) =b(j)/L(j,j);
end;
% Now we use this y to solve Rx = y
x = zeros( z, 1 );
for i=z:-1:1
x(i) = ( b(i) - R(i, :)*x )/R(i, i);
end
I put that into your code, and it works ;)
For helping you with pivot strategies it would be helpful to know what strategie you want/have to use as there are various versions. One simple version would be to just swap rows such that the diagonal element $a_{ii} neq 0$ for all $i = 1, dots, z$.
$endgroup$
add a comment |
$begingroup$
function[L R x]=LR2(A,b)
% This program will find a solution to
Ax=b using first giving the
decomposition of the matrix into L and
R and then solving.
% Part 1 - Is this matrix square and
nonsingular? give an error if not
[z y]=size(A);
if (z ~= y )
disp ( 'LR2 error: Matrix must be
square' );
return;
end;
if det(A)==0
disp('L singular error');
return;
end
% Part 2 : Decomposition of matrix
into L and R
L=zeros(z,z);
R=zeros(z,z);
for i=1:z
% Finding L
for k=1:i-1
L(i,k)=A(i,k);
for j=1:k-1
L(i,k)= L(i,k)-L(i,j)*R(j,k);
end
L(i,k) = L(i,k)/R(k,k);
end
% Finding R
for k=i:z
R(i,k) = A(i,k);
for j=1:i-1
R(i,k)= R(i,k)-L(i,j)*R(j,k);
end
end
end
for i=1:z
L(i,i)=1;
end
% Program shows R and L
R
L
% Now use a vector y to solve 'Ly=b'
y=zeros(z,1);
y(1)=b(1)/L(1,1);
for i=2:z
y(i)=-L(i,1)*y(1);
for k=2:i-1
y(i)=y(i)-L(i,k)*y(k);
y(i)=(b(i)+y(i))/L(i,i);
end;
end;
% Now we use this y to solve Rx = y
x=zeros(z,1);
x(1)=y(1)/R(1,1);
for i=2:z
x(i)=-R(i,1)*x(1);
for k=i:z
x(i)=x(i)-R(i,k)*x(k);
x(i)=(y(i)+x(i))/R(i,i);
end;
end
%print x
x
end
I have solved the original question. However there are still some problems with my solving of Ly=b and Rx=y. I also need to include pivoting. Any improvements would be greatly appreciated again!
$endgroup$
$begingroup$
It is easy to solve triangular systems by backward or forward substitutions. For pivoting you need some way to keep track of the permutations.
$endgroup$
– timur
Jan 9 '11 at 4:06
add a comment |
$begingroup$
After finding the L and U matrix, I tried to find the appropriate B matrix. After then, finding X is a little bit more easier than the traditional way.
% Modifying B
B_new = B;
for i = 2:n
for k = 1:i-1
B_new(i,1) = B_new(i,1) - B_new(k,1)*L(i,k);
end
end
% Finding X
x = zeros(n, 1);
i = n;
while(i>0)
x(i) = B_new(i,1);
k = n;
while(k > i)
x(i) = x(i) - x(k)*U(i,k);
k = k - 1;
end
x(i) = x(i) / U(i,i);
i = i - 1;
end
x
I hope it works for you :)
Greetings from Turkey
NOTE: n equals to z, B equals to b
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["$", "$"], ["\\(","\\)"]]);
});
});
}, "mathjax-editing");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "69"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
noCode: true, onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fmath.stackexchange.com%2fquestions%2f16838%2fimplement-a-program-in-matlab-for-lu-decomposition-with-pivoting%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
For backward and forward elimination I used
% Now use a vector y to solve 'Ly=b'
for j=1:z
for k=1:j-1
b(j)=b(j)-L(j,k)*b(k);
end;
b(j) =b(j)/L(j,j);
end;
% Now we use this y to solve Rx = y
x = zeros( z, 1 );
for i=z:-1:1
x(i) = ( b(i) - R(i, :)*x )/R(i, i);
end
I put that into your code, and it works ;)
For helping you with pivot strategies it would be helpful to know what strategie you want/have to use as there are various versions. One simple version would be to just swap rows such that the diagonal element $a_{ii} neq 0$ for all $i = 1, dots, z$.
$endgroup$
add a comment |
$begingroup$
For backward and forward elimination I used
% Now use a vector y to solve 'Ly=b'
for j=1:z
for k=1:j-1
b(j)=b(j)-L(j,k)*b(k);
end;
b(j) =b(j)/L(j,j);
end;
% Now we use this y to solve Rx = y
x = zeros( z, 1 );
for i=z:-1:1
x(i) = ( b(i) - R(i, :)*x )/R(i, i);
end
I put that into your code, and it works ;)
For helping you with pivot strategies it would be helpful to know what strategie you want/have to use as there are various versions. One simple version would be to just swap rows such that the diagonal element $a_{ii} neq 0$ for all $i = 1, dots, z$.
$endgroup$
add a comment |
$begingroup$
For backward and forward elimination I used
% Now use a vector y to solve 'Ly=b'
for j=1:z
for k=1:j-1
b(j)=b(j)-L(j,k)*b(k);
end;
b(j) =b(j)/L(j,j);
end;
% Now we use this y to solve Rx = y
x = zeros( z, 1 );
for i=z:-1:1
x(i) = ( b(i) - R(i, :)*x )/R(i, i);
end
I put that into your code, and it works ;)
For helping you with pivot strategies it would be helpful to know what strategie you want/have to use as there are various versions. One simple version would be to just swap rows such that the diagonal element $a_{ii} neq 0$ for all $i = 1, dots, z$.
$endgroup$
For backward and forward elimination I used
% Now use a vector y to solve 'Ly=b'
for j=1:z
for k=1:j-1
b(j)=b(j)-L(j,k)*b(k);
end;
b(j) =b(j)/L(j,j);
end;
% Now we use this y to solve Rx = y
x = zeros( z, 1 );
for i=z:-1:1
x(i) = ( b(i) - R(i, :)*x )/R(i, i);
end
I put that into your code, and it works ;)
For helping you with pivot strategies it would be helpful to know what strategie you want/have to use as there are various versions. One simple version would be to just swap rows such that the diagonal element $a_{ii} neq 0$ for all $i = 1, dots, z$.
edited Jun 2 '14 at 20:14
answered Jun 2 '14 at 20:08
TheWaveLadTheWaveLad
897720
897720
add a comment |
add a comment |
$begingroup$
function[L R x]=LR2(A,b)
% This program will find a solution to
Ax=b using first giving the
decomposition of the matrix into L and
R and then solving.
% Part 1 - Is this matrix square and
nonsingular? give an error if not
[z y]=size(A);
if (z ~= y )
disp ( 'LR2 error: Matrix must be
square' );
return;
end;
if det(A)==0
disp('L singular error');
return;
end
% Part 2 : Decomposition of matrix
into L and R
L=zeros(z,z);
R=zeros(z,z);
for i=1:z
% Finding L
for k=1:i-1
L(i,k)=A(i,k);
for j=1:k-1
L(i,k)= L(i,k)-L(i,j)*R(j,k);
end
L(i,k) = L(i,k)/R(k,k);
end
% Finding R
for k=i:z
R(i,k) = A(i,k);
for j=1:i-1
R(i,k)= R(i,k)-L(i,j)*R(j,k);
end
end
end
for i=1:z
L(i,i)=1;
end
% Program shows R and L
R
L
% Now use a vector y to solve 'Ly=b'
y=zeros(z,1);
y(1)=b(1)/L(1,1);
for i=2:z
y(i)=-L(i,1)*y(1);
for k=2:i-1
y(i)=y(i)-L(i,k)*y(k);
y(i)=(b(i)+y(i))/L(i,i);
end;
end;
% Now we use this y to solve Rx = y
x=zeros(z,1);
x(1)=y(1)/R(1,1);
for i=2:z
x(i)=-R(i,1)*x(1);
for k=i:z
x(i)=x(i)-R(i,k)*x(k);
x(i)=(y(i)+x(i))/R(i,i);
end;
end
%print x
x
end
I have solved the original question. However there are still some problems with my solving of Ly=b and Rx=y. I also need to include pivoting. Any improvements would be greatly appreciated again!
$endgroup$
$begingroup$
It is easy to solve triangular systems by backward or forward substitutions. For pivoting you need some way to keep track of the permutations.
$endgroup$
– timur
Jan 9 '11 at 4:06
add a comment |
$begingroup$
function[L R x]=LR2(A,b)
% This program will find a solution to
Ax=b using first giving the
decomposition of the matrix into L and
R and then solving.
% Part 1 - Is this matrix square and
nonsingular? give an error if not
[z y]=size(A);
if (z ~= y )
disp ( 'LR2 error: Matrix must be
square' );
return;
end;
if det(A)==0
disp('L singular error');
return;
end
% Part 2 : Decomposition of matrix
into L and R
L=zeros(z,z);
R=zeros(z,z);
for i=1:z
% Finding L
for k=1:i-1
L(i,k)=A(i,k);
for j=1:k-1
L(i,k)= L(i,k)-L(i,j)*R(j,k);
end
L(i,k) = L(i,k)/R(k,k);
end
% Finding R
for k=i:z
R(i,k) = A(i,k);
for j=1:i-1
R(i,k)= R(i,k)-L(i,j)*R(j,k);
end
end
end
for i=1:z
L(i,i)=1;
end
% Program shows R and L
R
L
% Now use a vector y to solve 'Ly=b'
y=zeros(z,1);
y(1)=b(1)/L(1,1);
for i=2:z
y(i)=-L(i,1)*y(1);
for k=2:i-1
y(i)=y(i)-L(i,k)*y(k);
y(i)=(b(i)+y(i))/L(i,i);
end;
end;
% Now we use this y to solve Rx = y
x=zeros(z,1);
x(1)=y(1)/R(1,1);
for i=2:z
x(i)=-R(i,1)*x(1);
for k=i:z
x(i)=x(i)-R(i,k)*x(k);
x(i)=(y(i)+x(i))/R(i,i);
end;
end
%print x
x
end
I have solved the original question. However there are still some problems with my solving of Ly=b and Rx=y. I also need to include pivoting. Any improvements would be greatly appreciated again!
$endgroup$
$begingroup$
It is easy to solve triangular systems by backward or forward substitutions. For pivoting you need some way to keep track of the permutations.
$endgroup$
– timur
Jan 9 '11 at 4:06
add a comment |
$begingroup$
function[L R x]=LR2(A,b)
% This program will find a solution to
Ax=b using first giving the
decomposition of the matrix into L and
R and then solving.
% Part 1 - Is this matrix square and
nonsingular? give an error if not
[z y]=size(A);
if (z ~= y )
disp ( 'LR2 error: Matrix must be
square' );
return;
end;
if det(A)==0
disp('L singular error');
return;
end
% Part 2 : Decomposition of matrix
into L and R
L=zeros(z,z);
R=zeros(z,z);
for i=1:z
% Finding L
for k=1:i-1
L(i,k)=A(i,k);
for j=1:k-1
L(i,k)= L(i,k)-L(i,j)*R(j,k);
end
L(i,k) = L(i,k)/R(k,k);
end
% Finding R
for k=i:z
R(i,k) = A(i,k);
for j=1:i-1
R(i,k)= R(i,k)-L(i,j)*R(j,k);
end
end
end
for i=1:z
L(i,i)=1;
end
% Program shows R and L
R
L
% Now use a vector y to solve 'Ly=b'
y=zeros(z,1);
y(1)=b(1)/L(1,1);
for i=2:z
y(i)=-L(i,1)*y(1);
for k=2:i-1
y(i)=y(i)-L(i,k)*y(k);
y(i)=(b(i)+y(i))/L(i,i);
end;
end;
% Now we use this y to solve Rx = y
x=zeros(z,1);
x(1)=y(1)/R(1,1);
for i=2:z
x(i)=-R(i,1)*x(1);
for k=i:z
x(i)=x(i)-R(i,k)*x(k);
x(i)=(y(i)+x(i))/R(i,i);
end;
end
%print x
x
end
I have solved the original question. However there are still some problems with my solving of Ly=b and Rx=y. I also need to include pivoting. Any improvements would be greatly appreciated again!
$endgroup$
function[L R x]=LR2(A,b)
% This program will find a solution to
Ax=b using first giving the
decomposition of the matrix into L and
R and then solving.
% Part 1 - Is this matrix square and
nonsingular? give an error if not
[z y]=size(A);
if (z ~= y )
disp ( 'LR2 error: Matrix must be
square' );
return;
end;
if det(A)==0
disp('L singular error');
return;
end
% Part 2 : Decomposition of matrix
into L and R
L=zeros(z,z);
R=zeros(z,z);
for i=1:z
% Finding L
for k=1:i-1
L(i,k)=A(i,k);
for j=1:k-1
L(i,k)= L(i,k)-L(i,j)*R(j,k);
end
L(i,k) = L(i,k)/R(k,k);
end
% Finding R
for k=i:z
R(i,k) = A(i,k);
for j=1:i-1
R(i,k)= R(i,k)-L(i,j)*R(j,k);
end
end
end
for i=1:z
L(i,i)=1;
end
% Program shows R and L
R
L
% Now use a vector y to solve 'Ly=b'
y=zeros(z,1);
y(1)=b(1)/L(1,1);
for i=2:z
y(i)=-L(i,1)*y(1);
for k=2:i-1
y(i)=y(i)-L(i,k)*y(k);
y(i)=(b(i)+y(i))/L(i,i);
end;
end;
% Now we use this y to solve Rx = y
x=zeros(z,1);
x(1)=y(1)/R(1,1);
for i=2:z
x(i)=-R(i,1)*x(1);
for k=i:z
x(i)=x(i)-R(i,k)*x(k);
x(i)=(y(i)+x(i))/R(i,i);
end;
end
%print x
x
end
I have solved the original question. However there are still some problems with my solving of Ly=b and Rx=y. I also need to include pivoting. Any improvements would be greatly appreciated again!
answered Jan 9 '11 at 1:32
Lucy MarshallLucy Marshall
1471212
1471212
$begingroup$
It is easy to solve triangular systems by backward or forward substitutions. For pivoting you need some way to keep track of the permutations.
$endgroup$
– timur
Jan 9 '11 at 4:06
add a comment |
$begingroup$
It is easy to solve triangular systems by backward or forward substitutions. For pivoting you need some way to keep track of the permutations.
$endgroup$
– timur
Jan 9 '11 at 4:06
$begingroup$
It is easy to solve triangular systems by backward or forward substitutions. For pivoting you need some way to keep track of the permutations.
$endgroup$
– timur
Jan 9 '11 at 4:06
$begingroup$
It is easy to solve triangular systems by backward or forward substitutions. For pivoting you need some way to keep track of the permutations.
$endgroup$
– timur
Jan 9 '11 at 4:06
add a comment |
$begingroup$
After finding the L and U matrix, I tried to find the appropriate B matrix. After then, finding X is a little bit more easier than the traditional way.
% Modifying B
B_new = B;
for i = 2:n
for k = 1:i-1
B_new(i,1) = B_new(i,1) - B_new(k,1)*L(i,k);
end
end
% Finding X
x = zeros(n, 1);
i = n;
while(i>0)
x(i) = B_new(i,1);
k = n;
while(k > i)
x(i) = x(i) - x(k)*U(i,k);
k = k - 1;
end
x(i) = x(i) / U(i,i);
i = i - 1;
end
x
I hope it works for you :)
Greetings from Turkey
NOTE: n equals to z, B equals to b
$endgroup$
add a comment |
$begingroup$
After finding the L and U matrix, I tried to find the appropriate B matrix. After then, finding X is a little bit more easier than the traditional way.
% Modifying B
B_new = B;
for i = 2:n
for k = 1:i-1
B_new(i,1) = B_new(i,1) - B_new(k,1)*L(i,k);
end
end
% Finding X
x = zeros(n, 1);
i = n;
while(i>0)
x(i) = B_new(i,1);
k = n;
while(k > i)
x(i) = x(i) - x(k)*U(i,k);
k = k - 1;
end
x(i) = x(i) / U(i,i);
i = i - 1;
end
x
I hope it works for you :)
Greetings from Turkey
NOTE: n equals to z, B equals to b
$endgroup$
add a comment |
$begingroup$
After finding the L and U matrix, I tried to find the appropriate B matrix. After then, finding X is a little bit more easier than the traditional way.
% Modifying B
B_new = B;
for i = 2:n
for k = 1:i-1
B_new(i,1) = B_new(i,1) - B_new(k,1)*L(i,k);
end
end
% Finding X
x = zeros(n, 1);
i = n;
while(i>0)
x(i) = B_new(i,1);
k = n;
while(k > i)
x(i) = x(i) - x(k)*U(i,k);
k = k - 1;
end
x(i) = x(i) / U(i,i);
i = i - 1;
end
x
I hope it works for you :)
Greetings from Turkey
NOTE: n equals to z, B equals to b
$endgroup$
After finding the L and U matrix, I tried to find the appropriate B matrix. After then, finding X is a little bit more easier than the traditional way.
% Modifying B
B_new = B;
for i = 2:n
for k = 1:i-1
B_new(i,1) = B_new(i,1) - B_new(k,1)*L(i,k);
end
end
% Finding X
x = zeros(n, 1);
i = n;
while(i>0)
x(i) = B_new(i,1);
k = n;
while(k > i)
x(i) = x(i) - x(k)*U(i,k);
k = k - 1;
end
x(i) = x(i) / U(i,i);
i = i - 1;
end
x
I hope it works for you :)
Greetings from Turkey
NOTE: n equals to z, B equals to b
answered Jan 17 at 17:10


Çağlayan DÖKMEÇağlayan DÖKME
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Mathematics Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fmath.stackexchange.com%2fquestions%2f16838%2fimplement-a-program-in-matlab-for-lu-decomposition-with-pivoting%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
w pN2F,k,Z
2
$begingroup$
You have assigned $L$ to be a zero matrix and the for loop for $i^{th}$ row of $L$ runs from the first column to the $(i-1)^{th}$ column. The entry $L(i,i)$ is still left unchanged. So the diagonal entries still remain zero.
$endgroup$
– user17762
Jan 8 '11 at 22:29
$begingroup$
@Sivaram so would it be "cheating" to simply assign all of my L(i,i)s to be 1? Thanks alot :)
$endgroup$
– Lucy Marshall
Jan 8 '11 at 22:32
1
$begingroup$
No it wouldn't. The best way is to decompose $L$ and $U$ directly in $A$. Since you know there will be ones on the diagonal, for huge matrix dimensions saving the previous known zeros and ones is a waste of memory space.
$endgroup$
– TheWaveLad
Jun 2 '14 at 20:18